初识 Django
Django 使用了类似 MVC 的架构,只是在定义和解释上略为不同,称为 MTV ( Model–Template–View )。
Django 处理 request 的流程:
- 浏览器送出 HTTP request
- Django 依据 URL configuration 分配至对应的 View
- View 进行资料库的操作或其他运算,并回传 HttpResponse
- 浏览器依据 HTTP response 显示网页画面
另外 Django 无需数据库就可以使用,它提供了对象关系映射器(ORM),可以使用 Python 代码来描述数据库结构。
Install
|
|
查看版本
Simple Example
Start project
|
|
目录结构:
各个文件的作用:
现在就可以在 mysite 目录下运行了
浏览器打开 ‘http://127.0.0.1:8000/‘ 能看到 “Welcome to Django” 的页面。
如果有别的程序占用端口,可以修改 django 运行的端口
|
|
修改 server 的 IP
修改 python 代码并保存时会自动加载 server,不用重新启动。然而,如果有添加文件等操作,就要重新启动了。
Create app
|
|
目录结构:
Projects vs apps
所谓 app 是指完成一些功能的 web 应用,比如博客系统(weblog system),公共记录的数据库(a database of public records)或者是一个简单的投票系统(a simple poll app)。project 是指一个特定网站的一系列配置文件和应用的集合。一个项目(project)可以包含多个应用(app),一个应用(app)可以被多个项目(project)使用。
Views
Django view 其实是一个 function,处理 HttpRequest,并回传 HttpResponse
- 收到 HttpRequest 参数
Django 从网页接收到 request 后,会将 request 中的信息封装产生一个 HttpRequest,并当成第一个参数,传入对应的 view function - 需要回传 HttpResponse
HttpResponse.content
HttpResponse.status_code …等
编辑 mysample/views.py:
这段代码做的是:
- 从 django.http 中引用 HttpResponse 类别
- 宣告 hello_world 这个 view
- 当 hello_world 被呼叫时,回传包含字串 Hello World! 的 HttpResponse
URL
Django 需要知道 URL 与 view 的对应关系,这个对应关系就是 URL conf (URL configuration),通常定义在 urls.py,包含了一连串的规则 (URL patterns),Django 收到 request 时,会一一比对 URL conf 中的规则,决定要执行哪个 view function
在 mysample 里新建一个 urls.py 文件来 map url,现在的目录结构:
编辑 mysample/urls.py:
现在要把这个 urls.py 连到 mysite 上,编辑 mysite/urls.py:
url() 函数接收四个参数,两个是必需的: regex 和 view,还有两个是可选的:kwargs 和 name。
- regex – 定义的 URL 规则
- 规则以 regular expression(正规表示式)来表达 - r'^mysample/' 代表的是 mysample/ 这种 URL - Django 从第一个开始,按顺序依次使用列表里的正则表达式来尝试匹配请求的 URL,直到遇到一个可以匹配的表达式 - 不尝试匹配 GET 或 POST 的参数,也不匹配域名部分
- view – 对应的 view function
- 当 Django 找到匹配的正则表达式时,就会调用这个视图函数 - 调用时传递的第一个参数是一个 HttpRequest 对象,后续的参数是所有被正则表达数捕捉的部分 - 如果正则式使用简单捕获,捕获结果会作为位置参数传递;如果使用名字捕获,捕获结果会作为关键字参数传递
- url() 参数之 kwargs
- 任意个关键字参数可以作为一个字典传递给目标视图函数
- url() 参数之 name
- 给 URL 起个名字,以便在 Django 的模板里无二义性的引用到它 - 这个非常有用的特性允许你只用更改一个文件就能全局性的改变某个 URL
我们使用的 include() 函数只是简单的引用其他的 URLconf 文件,让 URLconf 也能轻松的「即插即用」。请注意 include() 函数的正则表达式不包含 $ 符号(匹配字符串结尾)但是结尾有斜线。当 Django 遇到一个 include(),它砍掉被正则表达式匹配上的部分,并把剩余的字符串发送个作为参数的 URLconf 做进一步处理。
然后运行:
打开 ‘http://localhost:8000/mysample/',就可以看到 “Hello, world.” 主页。
Bootstrap
这里使用的模板是SB Admin
static 和 templates 文件
在 mysite 下新建文件夹 static,把 bootstrap 下载的模板复制到里面,然后在 mysite/mysite 下新建一个 templates 文件夹,把 html 文件复制到里面
目录结构:
注意要修改 html 里引用 css 和 js 的路径,如:
配置
修改 mysite 下的 settings.py,添加 templates 的目录路径以及 static files 的目录路径
渲染
修改 mysample/views.py,渲染 html 文件而不是仅仅输出一段文字
效果图
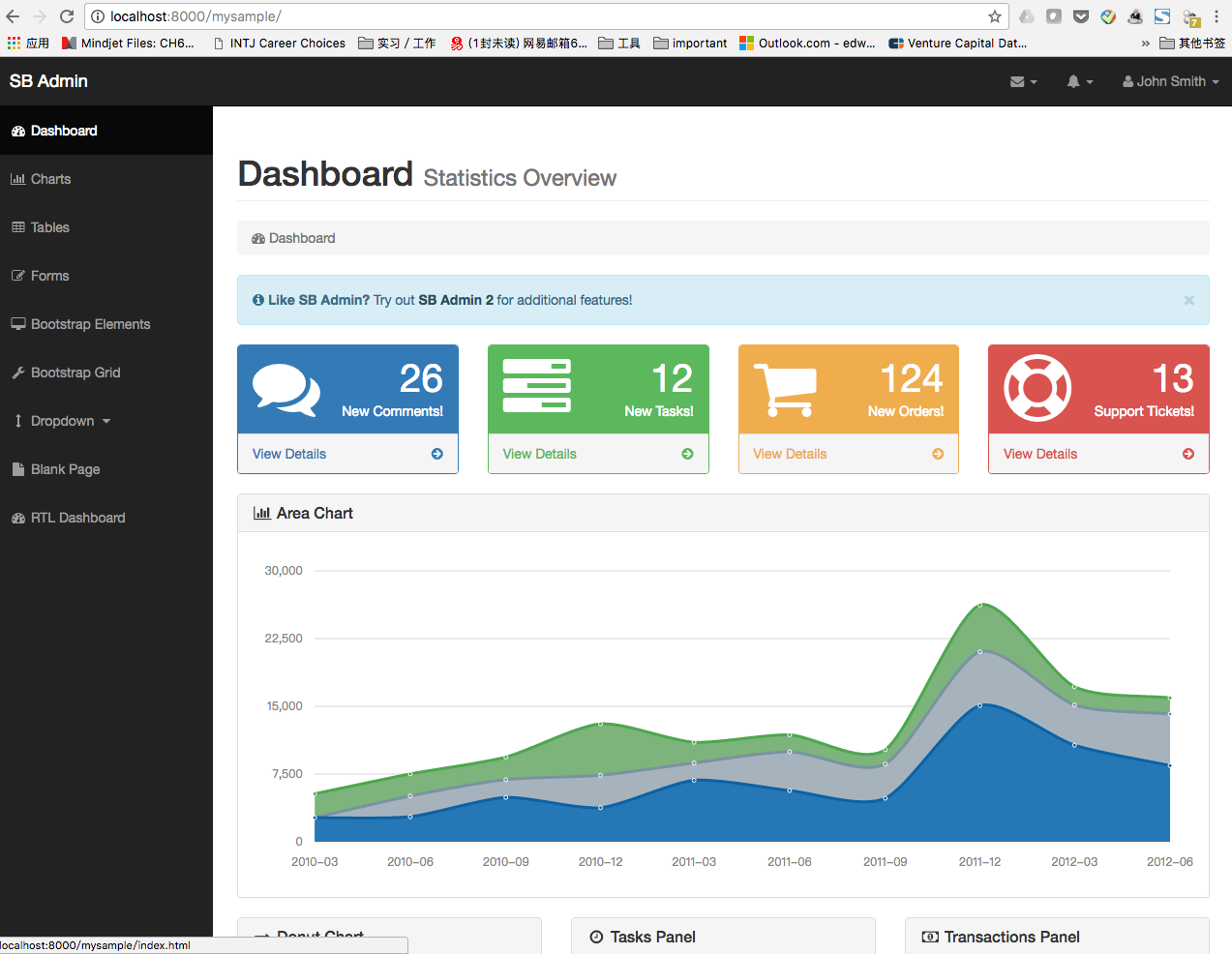